Functions
New Function Creation
The New Function interface in the Digisquares platform empowers developers to create, manage, and generate functions for various programming languages, such as TypeScript and JavaScript. This intuitive tool simplifies the process of function creation and integrates AI-powered assistance for generating the function body, managing parameters, and defining return types.
AI for Function Generation
The AI-assisted function generation feature allows users to describe the function they want to create in plain language, and the AI will generate the corresponding code for them. This feature accelerates the development process by automating routine code generation tasks.
Message Field:
- Enter a natural language instruction in the Message field to guide the AI in generating your function.
- Example: If you enter "Create a function that calculates the factorial of a number," the AI might generate:
function factorial(n: number): number {
if (n === 0) {
return 1;
}
return n * factorial(n - 1);
}
Send Button:
- After entering your description, click the Send button to submit the message. The AI will generate the function code based on your input. The generated function will appear in the code editor for review or modification.
- This feature saves time by allowing developers to focus on solving higher-level problems, while the AI handles the basic code generation.
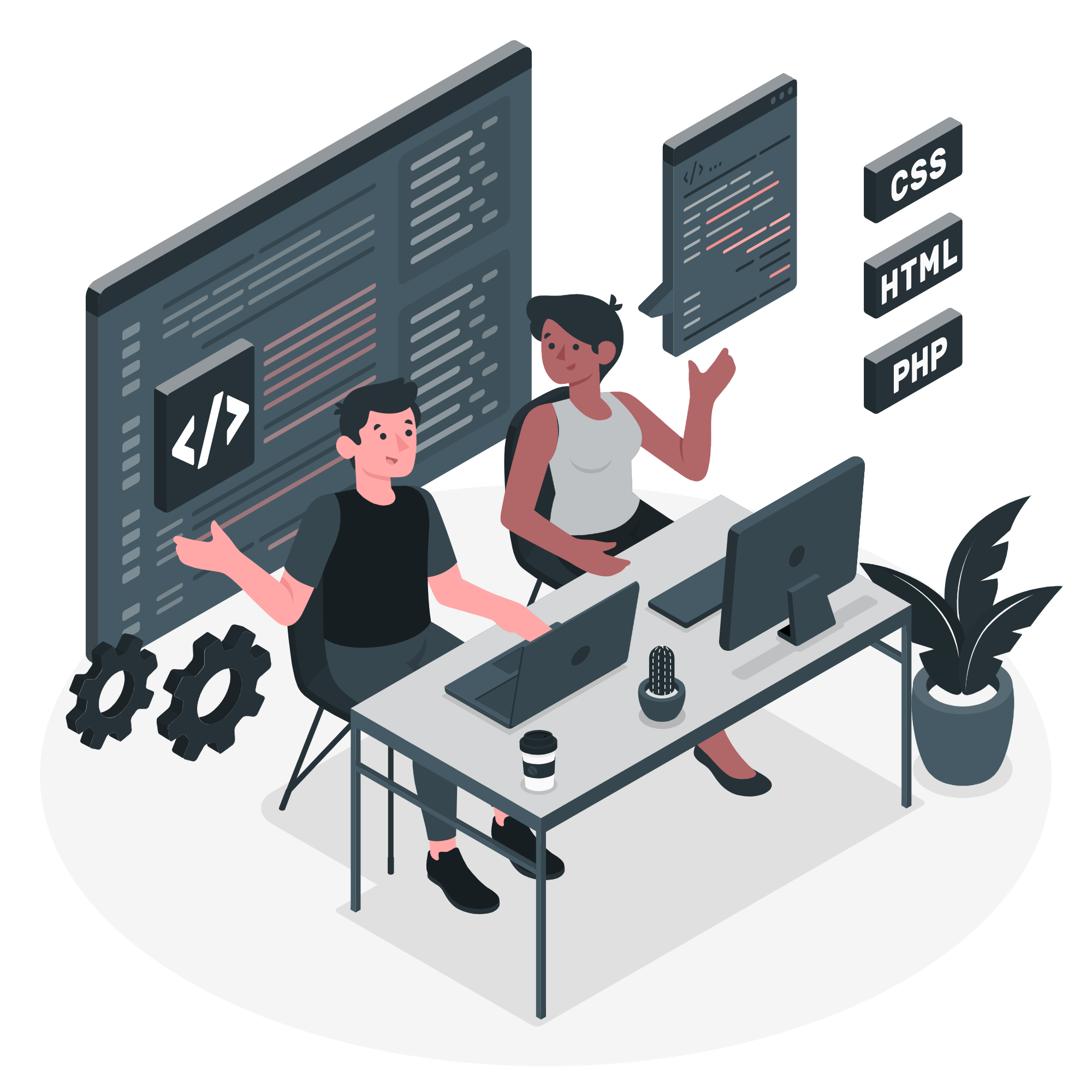
Main Features
-
Name Field:
- The Name field is used to assign a meaningful name to your function. This name should clearly reflect the purpose of the function, making it easy to identify in your codebase.
- Example: You might name your function
calculateSum
,fetchUserData
, etc.
-
Language Dropdown:
-
The Language dropdown allows you to choose the programming language for your function. Supported languages include TypeScript and JavaScript.
-
TypeScript: TypeScript provides static type checking, helping catch errors early and ensuring better code quality. When selected, the function will include type annotations.
-
JavaScript: JavaScript allows more flexibility with dynamic typing, making it suitable for rapid development.
-
Example: If you choose TypeScript, the function might look like:
function add(a: number, b: number): number {
return a + b;
}If you choose JavaScript, the function would look like:
function add(a, b) {
return a + b;
}
-
-
Params Button:
- Clicking the Params button opens an interface where you can define the parameters for your function. Parameters allow you to pass values into the function to customize its behavior.
- Example: For a function that adds two numbers, you might define two parameters
a
andb
.
-
Return Type Button:
- The Return Type button allows you to define the type of value your function will return. This is especially important in TypeScript where type safety is enforced.
- Example: For a function that calculates the sum of two numbers, the return type might be
number
in TypeScript.
-
NPM Button:
- The NPM button allows you to include Node Package Manager (NPM) packages within your function. This enables the use of third-party libraries in your code.
- Example: You can include libraries like
lodash
oraxios
for enhanced functionality.
-
Code Editor:
- The code editor is where you can view and edit the generated function code. It provides syntax highlighting based on the selected programming language (TypeScript or JavaScript) to improve readability.
- After the AI generates the function, you can make further modifications or write the function manually in the editor.
-
Save/Cancel:
- Once you are satisfied with the function, click Save to store it for future use or deployment.
- Click Cancel if you wish to discard the changes and return to the previous page.
Example Functions Visualizations
- Here are some example Fuctions visualizations created using Digisquares:
Using AI to generate functions significantly improves productivity by automating repetitive coding tasks. Developers no longer need to write basic code from scratch; instead, they can rely on the AI to generate clean, functional code. This allows for faster development cycles and reduced human error, ultimately streamlining the process of creating high-quality functions.
Summary
The New Function Creation interface in Digisquares offers a comprehensive, user-friendly environment for generating and managing functions in TypeScript and JavaScript. With AI-powered function generation, parameter and return type configuration, and the ability to integrate NPM packages, developers can create highly customizable and reusable functions efficiently.
This tool is ideal for both beginners and experienced developers, as it simplifies the function creation process while also providing flexibility for more complex requirements. By utilizing AI-assisted code generation, developers can accelerate their workflow and focus on higher-level problem-solving.